20 Common NestJS Errors: How to Avoid and Fix Them
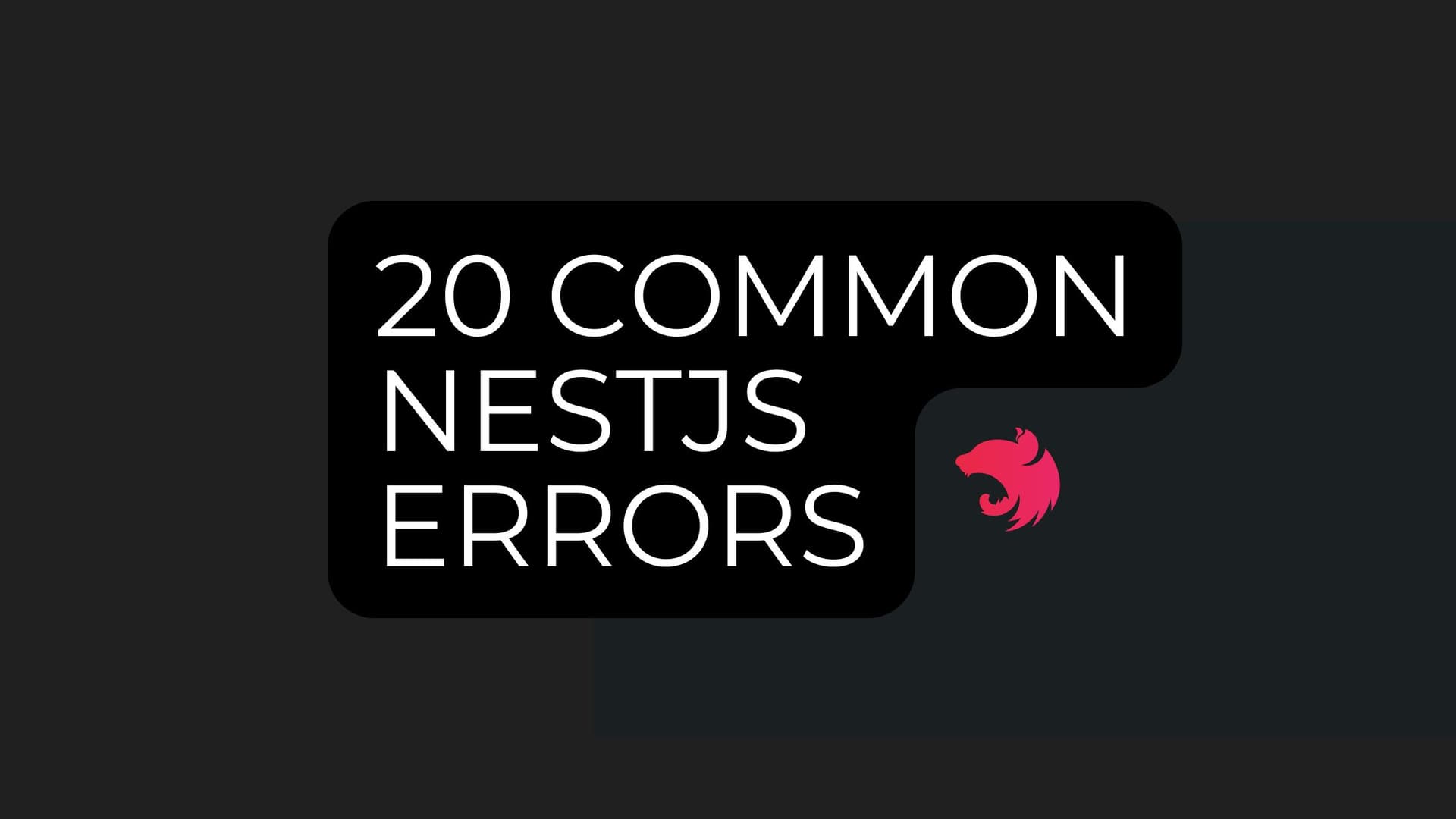
NestJS is a powerful and scalable framework for building server-side applications. However, like any framework, developers often encounter common pitfalls while working with it. In this guide, we will discuss 20 frequent errors when using NestJS, along with how to prevent and resolve them.
[1] Missing Dependencies Error
This error occurs when a required package is missing or not installed in your project. It often happens after cloning a project or when dependencies are accidentally removed.
Error Message:
Cannot find module 'some-package'
Cause:
This error occurs when a required package is missing or not installed
Solution:
Run the following command to install missing dependencies
npm install
If the error persists, try manually installing the missing package:
npm install some-package
[2] Module Not Found Error
This error occurs when a required module is not properly imported or registered in the NestJS application.
Error Message:
Error: Cannot find module 'someModule'
Solution:
Ensure that the module is correctly imported inside app.module.ts
:
import { SomeModule } from './someModule';
@Module({ imports: [SomeModule] })
export class AppModule {}
[3] Invalid Decorator Usage
This error occurs when a required module is not properly imported or registered in the NestJS application.
Error Message:
Error: Cannot find module 'someModule'
Solution:
Ensure that the module is correctly imported inside app.module.ts
:
import { SomeModule } from './someModule';
@Module({ imports: [SomeModule] })
export class AppModule {}
[4] Circular Dependency Error
This error occurs when a required module is not properly imported or registered in the NestJS application.
Error Message:
Error: Cannot find module 'someModule'
Solution:
Ensure that the module is correctly imported inside app.module.ts
:
import { SomeModule } from './someModule';
@Module({ imports: [SomeModule] })
export class AppModule {}
[5] Provider Not Found
This error occurs when a required module is not properly imported or registered in the NestJS application.
Error Message:
Error: Cannot find module 'someModule'
Solution:
Ensure that the module is correctly imported inside app.module.ts
:
import { SomeModule } from './someModule';
@Module({ imports: [SomeModule] })
export class AppModule {}
[6] Unrecognized Middleware
This error occurs when a required module is not properly imported or registered in the NestJS application.
Error Message:
Error: Cannot find module 'someModule'
Solution:
Ensure that the module is correctly imported inside app.module.ts
:
import { SomeModule } from './someModule';
@Module({ imports: [SomeModule] })
export class AppModule {}
[7] HTTP Exception Not Caught
This error occurs when a required module is not properly imported or registered in the NestJS application.
Error Message:
Error: Cannot find module 'someModule'
Solution:
Ensure that the module is correctly imported inside app.module.ts
:
import { SomeModule } from './someModule';
@Module({ imports: [SomeModule] })
export class AppModule {}
[8] Invalid DTO Validation
This error occurs when a required module is not properly imported or registered in the NestJS application.
Error Message:
Error: Cannot find module 'someModule'
Solution:
Ensure that the module is correctly imported inside app.module.ts
:
import { SomeModule } from './someModule';
@Module({ imports: [SomeModule] })
export class AppModule {}
[9] CORS Policy Issues
This error occurs when a required module is not properly imported or registered in the NestJS application.
Error Message:
Error: Cannot find module 'someModule'
Solution:
Ensure that the module is correctly imported inside app.module.ts
:
import { SomeModule } from './someModule';
@Module({ imports: [SomeModule] })
export class AppModule {}
[10] Incorrect Route Path
This error occurs when a required module is not properly imported or registered in the NestJS application.
Error Message:
Error: Cannot find module 'someModule'
Solution:
Ensure that the module is correctly imported inside app.module.ts
:
import { SomeModule } from './someModule';
@Module({ imports: [SomeModule] })
export class AppModule {}
Master Web Development: One Email at a Time!🔥
Subscribe to our newsletter for in-depth guides, project ideas, and best practices to enhance your coding journey, delivered straight to your inbox all for FREE. Your next project deserves to stand out!
Related Posts
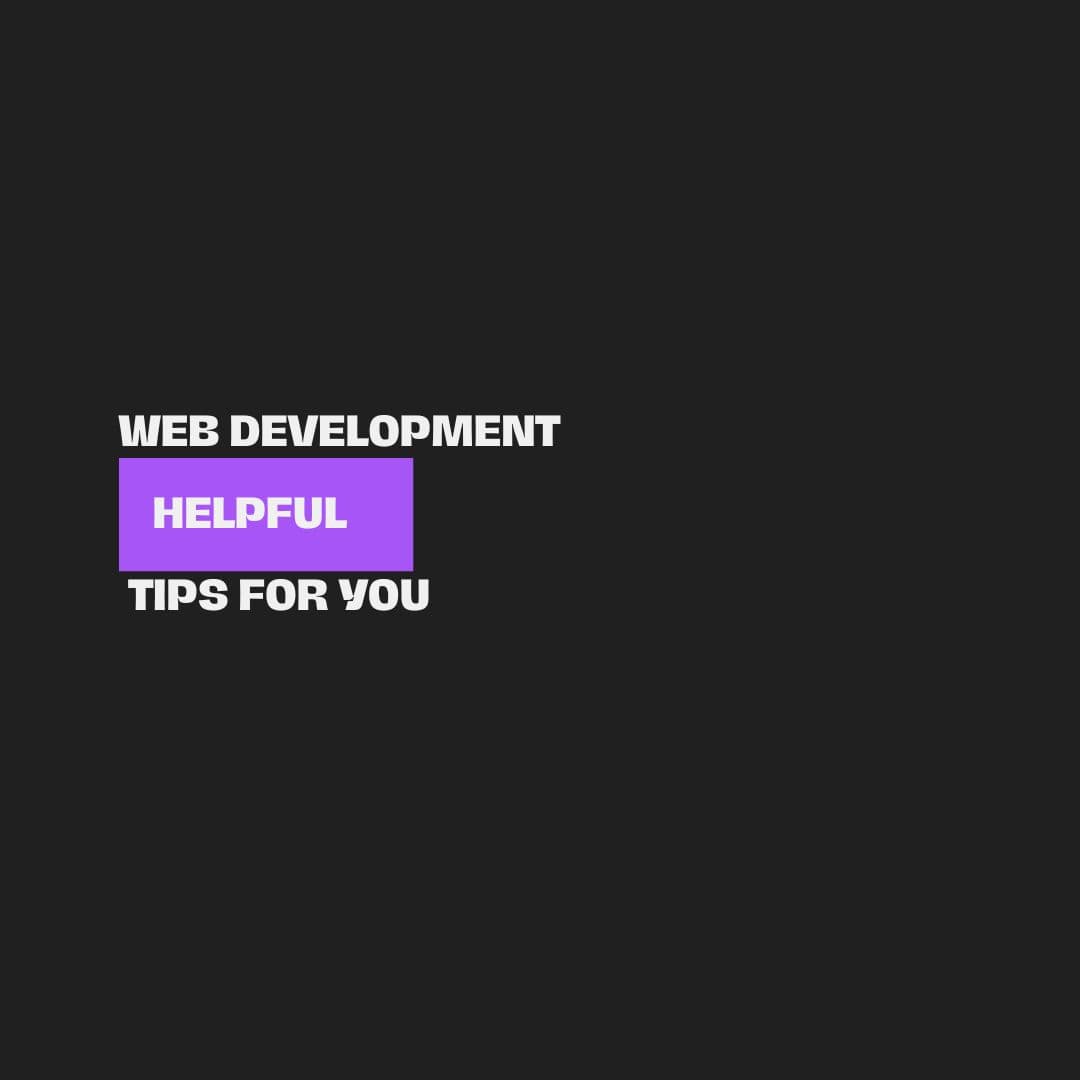
10 Essential Habits for Beginner Web Developers: Beyond Just Coding Skills
Starting out in web development? Discover the top 10 essential habits every beginner web developer should adopt beyond c....
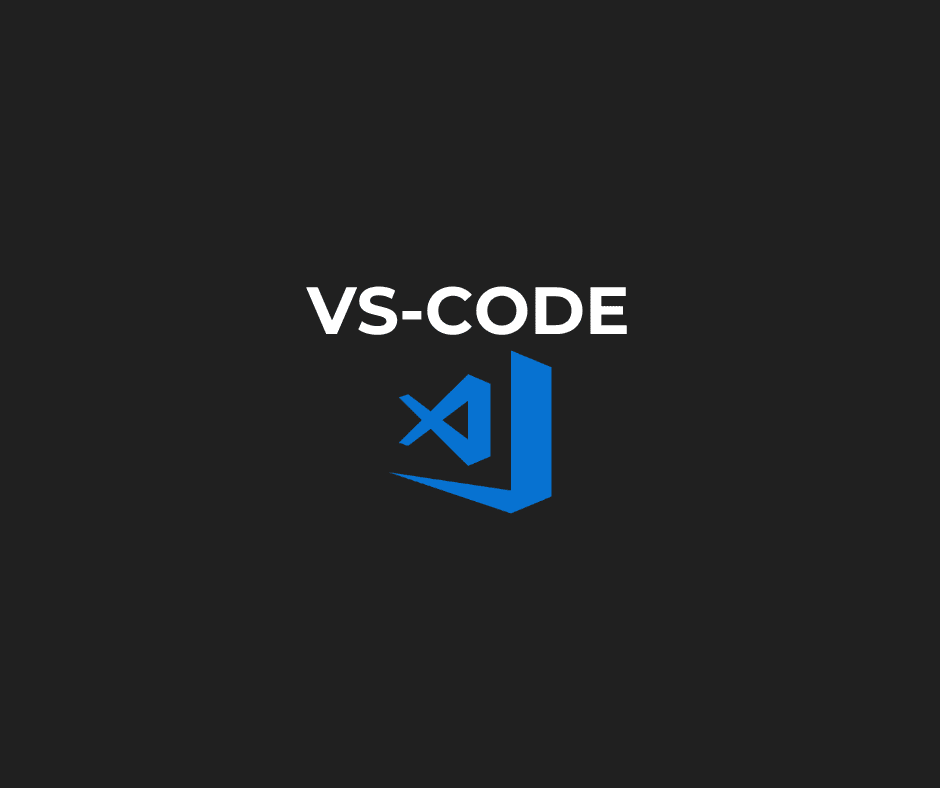
How to Download and Install VS Code: A Step-by-Step Guide for Complete Beginners
Learn how to download and install Visual Studio Code (VS Code) on your Windows, macOS, or Linux computer. This beginner-....